.
Introduction
In the realm of algorithmic trading, technical analysis plays a pivotal role in deciphering market trends and making informed investment decisions. One of the fundamental tools employed in technical analysis is the Simple Moving Average (SMA). The SMA calculates the average price of an asset over a specific period, providing a smoothed representation of its price movement.
Lumibot, a powerful Python library, simplifies the process of retrieving historical price data and applying technical analysis techniques. In this blog post, we will delve into how to effectively use Lumibot’s get_historical_prices function to obtain historical price data for an asset and subsequently calculate its Simple Moving Average.
Leveraging SMA for Effective Trading Strategies
The Simple Moving Average (SMA) is a versatile technical indicator that can be used to create various trading strategies. Here are five pointers on how to incorporate SMA into your trading bot:
1. Trend Following Strategies:
- Identify Uptrends: When the price consistently trades above the SMA, it suggests an uptrend. This indicates that the asset’s price is likely to continue rising. Your trading bot can use this signal to enter long positions.
- Identify Downtrends: Conversely, if the price consistently trades below the SMA, it suggests a downtrend, indicating a potential price decline. Your bot can use this signal to enter short positions.
Example: A trading bot might use a 50-day SMA and a 200-day SMA. If the 50-day SMA consistently trades above the 200-day SMA, it could indicate a bullish trend, prompting the bot to enter a long position.
2. Crossover Strategies:
- Golden Cross: A crossover occurs when a shorter-term SMA (e.g., 50-day) crosses above a longer-term SMA (e.g., 200-day). This is often interpreted as a bullish signal, suggesting a potential uptrend.
- Death Cross: A crossover occurs when a longer-term SMA crosses below a shorter-term SMA. This is often interpreted as a bearish signal, indicating a potential downtrend.
Example: A trading bot might use a 50-day SMA and a 200-day SMA. If the 50-day SMA crosses above the 200-day SMA (a Golden Cross), the bot could initiate a buy order, and if the 50-day SMA crosses below the 200-day SMA (a Death Cross), the bot could initiate a sell order.
3. Mean Reversion Strategies:
- Identify Overbought/Oversold Conditions: When the price deviates significantly from the SMA, it might indicate an overbought or oversold condition. An overbought condition suggests that the price is likely to fall, while an oversold condition suggests a potential price increase.
- Trading Signals: Your bot can use these signals to sell when the price is overbought and buy when the price is oversold, assuming that prices will eventually return to the average level.
Example: A trading bot might calculate the percentage difference between the current price and the 200-day SMA. If the difference is significantly positive (e.g., above 3 standard deviations), it might indicate an overbought condition, prompting the bot to sell.
4. Support and Resistance Strategies:
- Support Level: The SMA can act as a support level, especially during downtrends. If the price reaches the SMA and then bounces back up, it might signal a potential buying opportunity.
- Resistance Level: The SMA can also act as a resistance level, especially during uptrends. If the price reaches the SMA and then fails to break through, it might indicate a potential selling opportunity.
Example: A trading bot might monitor the price’s relationship to the 200-day SMA. If the price consistently tests the 200-day SMA as a support level during a downtrend and then bounces back up, the bot could initiate a buy order.
5. Combining SMA with Other Indicators:
- Enhanced Signal Reliability: Combining SMA with other technical indicators can help to improve the accuracy and reliability of trading signals.
Example: Combining SMA with the Relative Strength Index (RSI) can help to identify overbought and oversold conditions more effectively. If the price is above the SMA and the RSI is near the overbought level, it might suggest a potential sell signal.
Steps To Get the Simple Moving Average of the Historical Price of an Asset with Lumibot
Prerequisites
Must have Python installed in the system(version 3.10 or above)Install required Python packages.
pip install lumibot
Necessary imports for running the Python file.
from lumibot.strategies import Strategy
import pandas_ta as ta
Create ALPACA_CONFIG with API KEY and API SECRET by logging in or signing up at https://alpaca.markets/.………..
Steps for Using Simple Moving Average of the Historical Price of an Asset
Step 1: Add ALPACA_CONFIG Details
Alpaca is a broker, just like the interactive broker. The details below are required to use the Alpaca broker API.
ALPACA_CONFIG = {
"API_KEY": "YOUR_API_KEY_HERE", # Get your API Key from
https://alpaca.markets/
"API_SECRET": "YOUR_SECRET_HERE", # Get your secret from
https://alpaca.markets/
"PAPER":True # Set to False for real money
}
Step 2: Create a GetHistoricalPrice Class
Once you have added the Alpaca config detail, create a GetHistoricalPrice class, which will inherit the Strategy class as below.
class GetHistoricalPrice(Strategy):
Step 3: Add on_trading_iteration() Method
Once you have added the initialize method, follow with the creation of on_trading_iteration() method as below:
def on_trading_iteration(self):
# Retrieve historical prices
bars = self.get_historical_prices("AAPL", 10, "day")
df = bars.df
# Calculate the 10-day Simple Moving Average (SMA)
df['SMA_10'] = ta.sma(df['close'], length=10)
# Log a message if the last SMA value is greater than 20
if df['SMA_10'].iloc[-1] > 200:
self.log_message("SMA is more than 200")
else:
self.log_message("SMA is less than or equal to 200")
# Print the DataFrame for debugging
print(df)
The provided code snippet defines a function that retrieves historical price data for AAPL, calculates a 10-day Simple Moving Average (SMA), and prints the resulting DataFrame. This function could be used to analyze market trends, identify potential trading opportunities, or backtest trading strategies.
Note 1: Running the Code in the Same File
In Python, if __name__ == “__main__”: is a conditional statement, which allows you to control the execution of code depending on whether the script is run directly or imported as a module. This implies that the code will run only if runs as a script and not as a module.
if __name__ == "__main__":
Step 4: Import Alpaca and Trader
Import Alpaca and Trader classes from Lumibot.brokers and Lumibot.traders modules. While Alpaca is an interface to the Alpaca trading platform, it leverages us with the functionalities to interact with the Alpaca API for implementing things like placing orders, managing positions, and fetching market data like Historical Price Data, which we are doing in this blog.
The Trader class helps orchestrate the trading process, managing multiple trading strategies, interacting with brokers like Alpaca, Interactive Brokers, and Tradiers, handling order execution and position management, and ensuring a framework for live trading and backtesting.
from lumibot.brokers import Alpaca
from lumibot.traders import Trader
Step 5: Create Trader Class Object
As you import the Alpaca and Trader class, create the trader object of the Trader() class. This object will be responsible for handling trading strategies and interactions with the Alpaca API. It can potentially execute trades, analyze market data, and implement various trading algorithms.
trader = Trader()
Step 6: Create an Object of Alpaca Class
On creation of the trader class object, create the object of the Alpaca class by passing the Alpaca_Config array created above.
broker = Alpaca(ALPACA_CONFIG)
Step 7: Create an Object of GetHistoricalPrice Class
Once we have created the object for the Alpaca class, we will create an object of the GetHistoricalPrice class by passing the Alpaca object (broker) as a parameter to the GetHistoricalPrice class.
strategy = GetHistoricalPrice(broker=broker)
Step 8: Pass the Strategy to the Trader Class Object
On creation of the object of the GetHistoricalPrice class, add the strategy to the trader class object using the add_strategy() method.
trader.add_strategy(strategy)
Step 9: Start the Overall Trading Process
The code below starts the overall trading process. This typically executes backtesting or a live trading process for a collection of strategies within a trading platform. This command starts the execution engine. It establishes the connection with a broker, which is Alpaca, and starts background tasks like market data ingestion and order management. Briefly, it is the starting point of the trading process.
trader.run_all()
Complete Code
from lumibot.strategies import Strategy
import pandas_ta as ta
ALPACA_CONFIG = {
"API_KEY": "",
"API_SECRET": "",
"PAPER": True
}
class GetHistoricalPrice(Strategy):
def on_trading_iteration(self):
# Retrieve historical prices
bars = self.get_historical_prices("AAPL", 10, "day")
df = bars.df
# Calculate the 10-day Simple Moving Average (SMA)
df['SMA_10'] = ta.sma(df['close'], length=10)
# Log a message if the last SMA value is greater than 20
if df['SMA_10'].iloc[-1] > 200:
self.log_message("SMA is more than 200")
else:
self.log_message("SMA is less than or equal to 200")
# Print the DataFrame for debugging
print(df)
if __name__ == "__main__":
from lumibot.brokers import Alpaca
from lumibot.traders import Trader
trader = Trader()
broker = Alpaca(ALPACA_CONFIG)
strategy = GetHistoricalPrice(broker=broker)
trader.add_strategy(strategy)
trader.run_all()
Output
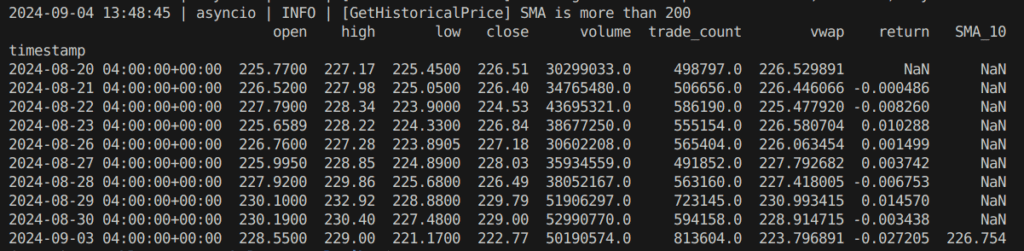
Conclusion
The Simple Moving Average (SMA) is a versatile technical indicator that can be effectively used in trading strategies. By following the steps outlined in this guide, you can retrieve historical price data using Lumibot, calculate the SMA, and leverage it to identify trends, generate trading signals, and make informed investment decisions. Lumibot simplifies the process of retrieving historical price data and calculating the SMA, making it a valuable tool for traders of all levels. The SMA provides valuable insights into market trends, support and resistance levels, and potential trading opportunities. Combining SMA with other technical indicators can further enhance the accuracy and reliability of trading signals. Start your algorithmic trading journey today by incorporating the SMA into your trading strategies using Lumibot.